Problem: Write a Java program to check whether the given number is Twisted Prime or not.
A number is said to be Twisted Prime if it is a prime number and its reverse is also a prime number.
Example:
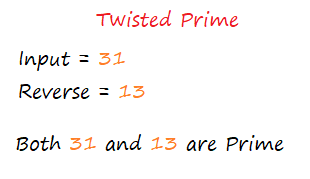
Steps to check Twisted Prime in Java:
- Input a number.
- Check if the number is prime.
- If the number is prime, compute its reverse.
- Check if the reverse is prime.
- If both step-2 and step-4 are true, then the input number is Twisted Prime, else not.
Here is the implementation of the steps in Java:
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner in=new Scanner(System.in);
System.out.print("Enter a number: ");
int n=in.nextInt();
if(isPrime(n)){
//Computing Reverse
int rev=0;
while(n>0){
rev=rev*10 + n%10;
n=n/10;
}
if(isPrime(rev))
System.out.println("Twisted Prime");
else
System.out.println("Not a Twisted Prime");
}
else
System.out.println("Not a Twisted Prime");
}
//Function to Check Prime Number
private static boolean isPrime(int n){
for(int i=2; i<(n/2); i++){
if(n%i==0)
return false;
}
return true;
}
}
Output:
Enter a number: 31 Twisted PrimeIn the above program, the isPrime(int n)
function returns true
if the passed number (i.e. n
) is a prime number, otherwise it returns false
.
We use the isPrime(int n)
to check whether the input and its reverse are prime or not.
If anyone of them is not a prime number then it means input is not a Twisted Prime.
If both the numbers are prime then the input is said to be a Twisted Prime number.
In this programming example, we learned to check Twisted Prime number in Java programming language.