Summary: In this tutorial, we will learn different ways to convert a decimal number into its corresponding binary representation in C programming language.
Example:
Input: 1111 Output: 15 Input: 101 Output: 5
Method 1: Using While Loop
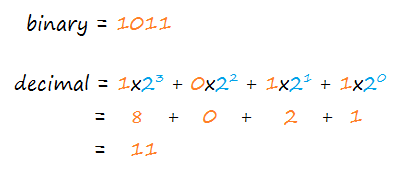
This is the standard way of converting a binary number to its corresponding decimal representation.
We multiply each binary digit with their corresponding exponential value of 2 (i.e. 2position_from_right -1) and sum them up.
In our program, we use a while loop to extract each binary digit, multiply them with 2x and sum them.
#include <stdio.h>
int main()
{
int bin, i=0, decimal = 0, digit;
printf("Enter a Binary number: \n");
scanf("%d",&bin);
while(bin != 0){
//extract the digit
digit = bin%10;
//multiply the digit with the 2x and add to the decimal
decimal = decimal + pow(2,i) * digit;
//remove the digit from the binary
bin = bin/10;
//increment the exponent power
i = i+1;
}
printf("Decimal: %d", decimal);
return 0;
}
Output:
Enter a Binary number:1011
Decimal: 11
Method 2: Using Recursion
We can also convert a binary number into a decimal using recursion.
In this method, we use the same approach as the above program but implement it in a recursive way.
#include <stdio.h>
//recursive function to convert binary to decimal
int binToDec(int bin, int i){
/*
*i represents the nth recursive call as well as
*nth digit from right, so it can be used as an exponent
*/
//base condition
if(bin == 0 || bin == 1)
return pow(2,i)*bin;
//extract binary digit, then multiply 2x and-
//sum with the next recusive call
int digit = bin%10;
return (pow(2,i) * digit) + binToDec(bin/10, ++i);
}
int main()
{
int bin, decimal;
printf("Enter a Binary number: \n");
scanf("%d",&bin);
decimal = binToDec(bin,0);
printf("Decimal: %d", decimal);
return 0;
}
Output:
Enter a Binary number:101
Decimal: 5
In each recursive call, we extract a digit from the binary number and multiply it with its corresponding value of 2 raised to the power its position in the original binary number from the right.
Finally, we sum the multiplication result with the next recursive call result.
Note: Is it important to eliminate the last digit from binary on every recursive call.
In this programming example, we learned to convert a binary number into decimal in C. If you have any doubts or suggestions then please comment below.