Summary: In this example, we will learn different ways to reverse a string in the C programming language.
Example:
If the user enters ‘Pencil‘, then the output should be ‘licneP‘.
Input: Pencil Output: licneP
Method 1: Using Loop
The idea is to assign characters of the input string to its reverse in reverse order using the ‘while’ or ‘for’ loop.
#include <stdio.h>
int main()
{
char str[30];
char rev[30];
int i=0, l=0;
printf("Enter a string \n");
scanf("%[^\n]s",str);
//computing length of the 'str'
for(l=0; str[l] != '\0'; ++l);
//assign characters to 'rev' in reverse order
while(--l>=0){
rev[i++] =str[l];
}
rev[i]='\0';
printf("\n");
printf("String: \t %s \n",str);
printf("Reverse: \t %s",rev);
return 0;
}
Output:
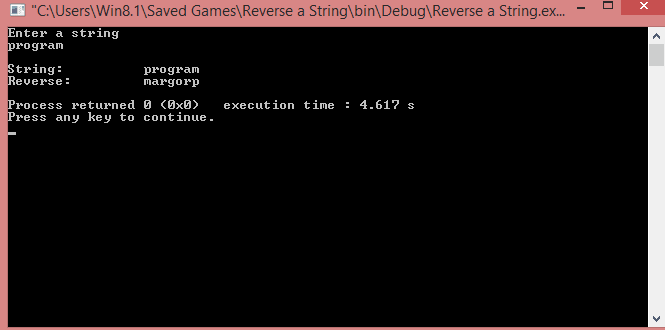
Using this method, we can also reverse a string with whitespace i.e., a whole sentence.
Method 2: Using Recursion
We can also reverse a string in C using the recursion algorithm.
We extract an character from the string on each recursive call and reassign them in reverse order during the traceback of the recursive calls.
#include <stdio.h>
//recursive function
void reverse(char *str, int i, int length){
//Base condition - when index exceeds the string length
if(i == length)
return;
//extract character
char ch = str[i];
//recusively call for next character
reverse(str, i+1, length);
//reassign in reverse order
str[length-i-1] = ch;
}
int main()
{
char str[30];
int length =0;
printf("Enter a string \n");
scanf("%[^\n]s",str);
//computing length of the 'str'
for(length=0; str[length] != '\0'; ++length);
//reverse using the recusive function
reverse(str, 0, length);
//output the reversed string
printf("%s",str);
return 0;
}
Output:
Enter a stringadarsh
hsrada
Method 3: Using strrev()
string.h
library has an inbuilt function called strrev()
which in-place reverses the string passed as an argument.
Note: strrev() only works with non-linux compiler. strrev() method is not present in glibc compiler.
#include <stdio.h>
#include <string.h>
int main()
{
char string[30];
printf("Enter a string \n");
scanf("%[^\n]s",string);
printf("\n");
printf("String: \t %s \n",string);
//reversing string using inbuilt strrev() method
strrev(string);
printf("Reverse: \t %s",string);
printf("\n");
return 0;
}
Output:
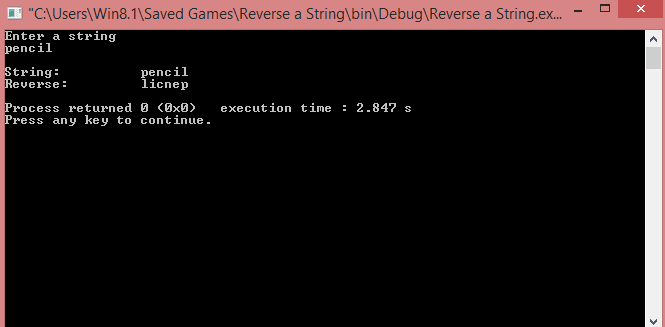
These are three different ways we can use to reverse a string in a C programming language. Please comment below if you know any other methods.