Problem: Write a C program to read and print students’ details using structure.
We will use an array of structure objects to store the details of multiple students in C.
Each Structure (i.e. Student) will have:
- Name
- Roll
- Stream
For the demonstration purpose, we will read 2 student’s details, store them in the array, and finally print it.
#include <stdio.h>
typedef struct{
char name[30];
int roll;
char stream[30];
} Student;
int main()
{
char buffer;
//number of students
int n=2;
//array of students (i.e. structure array)
Student students[n];
//Taking each student details as input
printf("Enter %d Student Details \n \n",n);
for(int i=0; i<n; i++){
printf("Student %d:- \n",i+1);
printf("Name: ");
scanf("%[^\n]",students[i].name);
scanf("%c",&buffer);
printf("Roll: ");
scanf("%d",&students[i].roll);
scanf("%c",&buffer);
printf("Stream: ");
scanf("%[^\n]",students[i].stream);
scanf("%c",&buffer);
printf("\n");
}
//display student details
printf("-------------- All Students Details ---------------\n");
for(int i=0; i<n; i++){
printf("Name \t: ");
printf("%s \n",students[i].name);
printf("Roll \t: ");
printf("%d \n",students[i].roll);
printf("Stream \t: ");
printf("%s \n",students[i].stream);
printf("\n");
}
return 0;
}
Output:
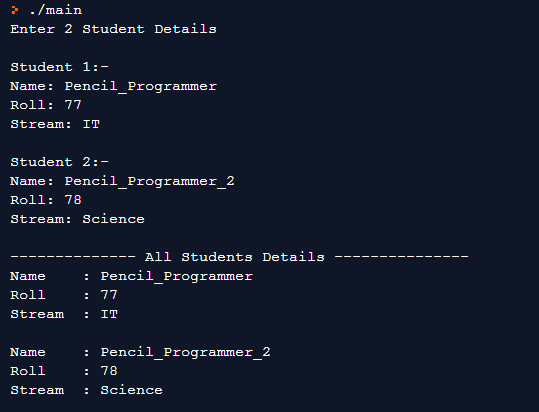
Student students[n]
is the array declaration of the Student
type. It means each element in the array holds the details of a particular student.
The value of n
determines the number of students details the array can have.
Using the ‘for’ loop, we input the details of n
students and output the same in the structured format.
Note: After every input, we have used scanf("%c", &buffer)
to catch automatic insertion of the null
value.
In this programming example, we learned to read and store students’ details using the structure in C. Comment below your suggestions.
if i also put a code where the user inputs a grade for the students where 75 is the passing grade, what code will i use to display the highest grade, number of students failed and number of students passed?