Summary: In this tutorial, we will learn what memory leak is, when it occurs and how can we avoid it in C++.
What is a Memory leak?
When we use a new operator on raw pointers in our program, we are actually allocating memory for it in the heap.
This memory space in the heap remains occupied until the object in the heap is deleted.
The ideal point for freeing this space is when the pointer is no longer in use or is out of scope.
If we don’t do so and the pointer is destroyed, the space in the heap remains occupied, causing a memory leak.
For example: Suppose, we have a class in our C++ program that has a raw pointer as one of its properties:
class Myclass{
public:
int *p;
Myclass (int n){
p = new int {n}; //Allocating space in heap, needs to be free when not used
}
};
We create an object of the class which will be automatically be destroyed at the end of the program or scope.
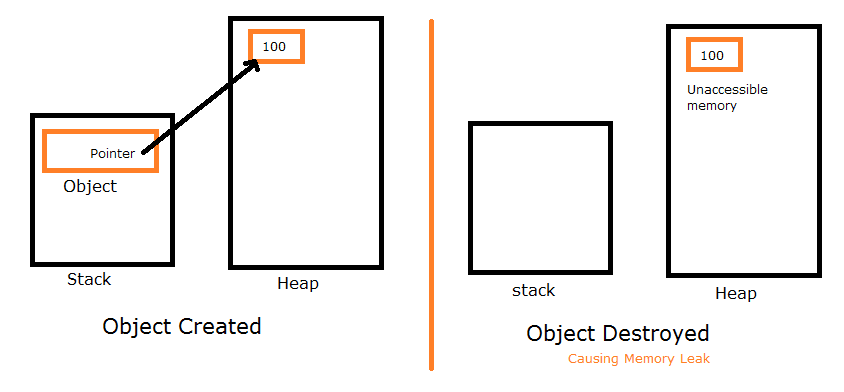
But what’s to be noted is that the memory which we’ve used for the pointer is still containing data but can no longer be accessed (because the object is detroyed).
This inaccessible memory is termed as a leak of memory.
Although it does not have much impact in terms of space, it could be, if it were a list that had the ability to grow automatically.
Hence taking care of such leaks is very important to maintain the efficiency of our programs.
How to Avoid Memory Leak?
There are two possible ways to avoid memory leak in C++:
- By manually deleting the pointers.
- By using smart pointers.
The best place to manually delete a pointer using the delete
keyword is in the destructor of the class.
delete
keyword in C++ deallocates the memory allocated to a pointer object (by thenew
keyword) in the heap.
The destructor of the class is called whenever its object is destroyed or goes out of scope.
Here is the example that appropriately deletes the pointer (allocated memory) to avoid memory leak:
#include <iostream>
using namespace std;
class Myclass{
public:
int *p;
//Constructor
Myclass (int n){
cout << "Allocating Space"<< endl;
p = new int {n};
}
void displayData(){
cout << "Data is: "<< *p <<endl;
}
//Destructor
~Myclass(){
cout << "Deleting pointer" << endl;
delete p;
}
};
int main()
{
Myclass obj(100);
obj.displayData();
}
Output:
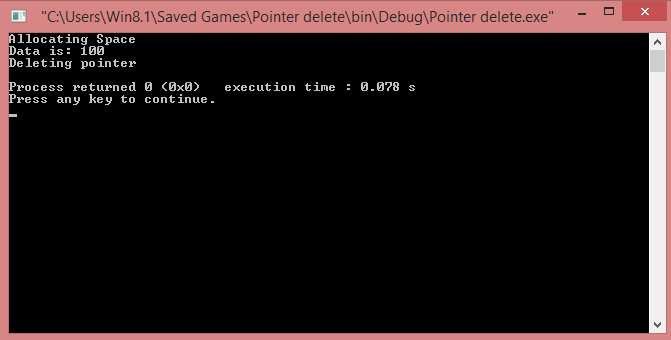
Why Smart Pointers should be preferred over Raw Pointers?
In case of raw pointers, to avoid memory leaks, we have to manually delete them inside the destructor of the class.
It is best to use smart pointers in such cases because we don’t need to explicitly free up the allocated space in the heap.
Smart pointers itself deallocates the allocated space, when the object containing it is destroyed.