Problem: Write a Program in Python to convert a decimal number into its corresponding octal representation.
Example:
Input: 8 Output: 10 Input: 15 Output: 17
Convert Decimal to Octal in Python using Loop
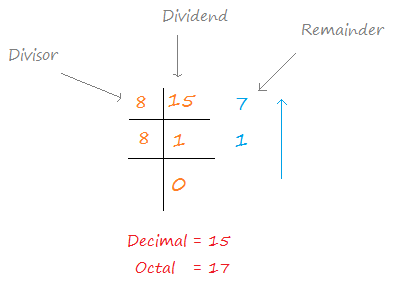
The standard way to convert a decimal to an octal is to divide the decimal by 8 until it reduces to 0.
After the division is over, if we stack the remainders in a bottom-up manner the result value will be the equivalent octal number.
decimal = int(input("Enter a decimal number: "))
octal = 0
ctr = 0
temp = decimal #copying number
#computing octal using while loop
while(temp > 0):
octal += ((temp%8)*(10**ctr)) #Stacking remainders
temp = int(temp/8) #updating dividend
ctr += 1
print("Binary of {x} is: {y}".format(x=decimal,y=octal))
Output:
Enter a decimal number: 15
Binary of 15 is: 17
Convert Decimal to Octal in Python using Recursion
To convert decimal into octal using recursion, we pass the quotient (dividend/8) to the next recursive call and output the remainder value (dividend%8).
We repeat the process until the number reduces to zero (i.e. until decimal > 0).
Since recursion implements stack, the remainders get printed in a bottom-up manner and we get the equivalent octal number.
def dectoOct(decimal):
if(decimal > 0):
dectoOct((int)(decimal/8))
print(decimal%8, end='')
decimal = int(input("Enter a decimal number: "))
print("Octal: ", end='')
dectoOct(decimal)
Output:
Enter a decimal number: 8
Octal: 10
Convert Decimal to Octal using oct()
The built-in Python method oct()
method returns the octal representation of the decimal number passed as a parameter.
It returns an octal number in the form of 0oxyz
, where xyz
is the actual octal number value.
>>> print(oct(15))
0o17
Comment below your doubts or suggestions if you have any.