Summary: In this tutorial, we will learn to fetch and post tweets on Twitter using API in Python.
Prerequisites: An active account on Twitter.
Step 1: Create a Twitter Developer Account
We need API Key and Access Token to programmatically access our Twitter account. For this, we must have a Twitter developer account.
So apply here using your Twitter credentials to create a Twitter developer account.
Step 2: Create an App in Developer Account
After the account has been created and verified, create an app by going here.
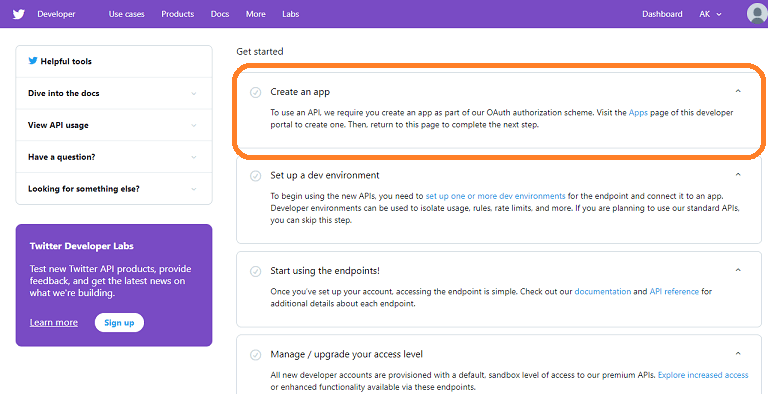
Provide the information required to create the new app. After this, we can now access the API tokens to integrate into our project (in our case is a Python script).
Step 3: Get the API Key and API Token
Select your newly created app and go to the ‘keys and tokens’ section to get your API keys.
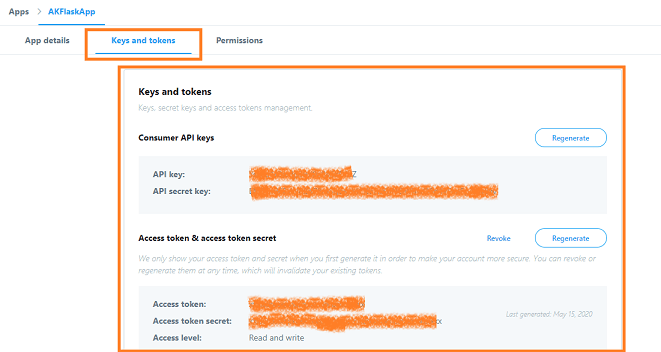
Copy all API Keys and tokens to use in your code.
Step 4: Install Python-Twitter Library
To access our Twitter account, we will use a 3rd party library python-twitter. Install it using the following command in PC Terminal or Command Prompt.
$ pip install python-twitter
Step 5: Use Python-Twitter to Fetch Tweets
After successfully installing the library, paste your API keys and tokens details into the respective fields of the following script:
import twitter
#paste your API keys and token here
consumer_key = 'your API Key'
consumer_secret = 'your API secret key'
access_token = 'your ACCESS Token'
access_token_secret = 'your ACCESS Token Secret'
api = twitter.Api(consumer_key=consumer_key,
consumer_secret=consumer_secret,
access_token_key=access_token,
access_token_secret=access_token_secret)
#fetch all tweets in your timeline and output them.
all_tweets = api.GetHomeTimeline()
print([s.text for s in all_tweets])
#fetch your tweets and output them.
my_tweets = api.GetUserTimeline(api.VerifyCredentials().id)
print([s.text for s in my_tweets])
On carefully implementing all the steps, you will be able to see your tweets as output.
Post Tweets using Python
Using the same API object, we can post a tweet from our python code as follows:
tweet = "This is my next Tweet!"
api.PostUpdate(tweet)
Just call the postUpdate()
method passing the tweet text as a parameter.
Using the twitter API we can also see the latest twitter trends of different countries around the globe.
Refer to python-twitter documentation, to know more about the use of the Twitter API.