Summary: In this tutorial, we will learn 5 different types of inheritance in Python with the help of examples.
Inheritance is one of the features of the Python language that allows us to reuse the code of the existing classes.
For example, in the following code, we are inheriting the class A
in class B
and doing so allows us to reuse the display
method of class A
in class B
:
class A:
def display(self):
print("Hello")
class B(A):
def display(self):
super().display()
print("World")
Here we have inherited only a single class. If we need to inherit more classes to keep our code DRY, we can do so.
It is because Python supports different types of inheritance.
On the basis of number of child classes and pattern of inheritance, we can classify inheritance in Python into 5 major types:
- Single Inheritance
- Multiple Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Let’s see an example of each type.
1. Single Inheritance
In Single inheritance, one class inherits another single class. It is the very basic inheritance where a single child class inherits the properties and functions of a parent class.
The following block diagram illustrates single inheritance in Python:
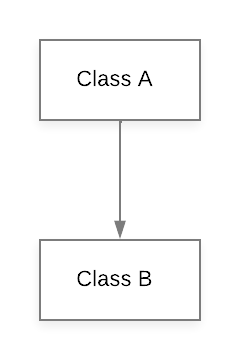
Inheritance is referred to as ‘IS A’ relationship, implying that one class should only inherit other if there is a ‘IS A’ relationship between them.
Example: Dog is an Animal.
By inheriting class A
in class B
, we can access the properties and function of class A in class B using the super() method.
class A:
def display(self):
print("Hello")
class B(A):
def display(self):
super().display()
print("World")
b = B()
b.display()
Output:
Hello
World
2. Multiple Inheritance
In multiple inheritance, one class inherits multiple other classes. In this, the child class has access to the methods and properties of all its parent classes.
Programming langugages such as Java does not allow multiple inheritance.
The following block diagram illustrates an example of multiple inheritance:
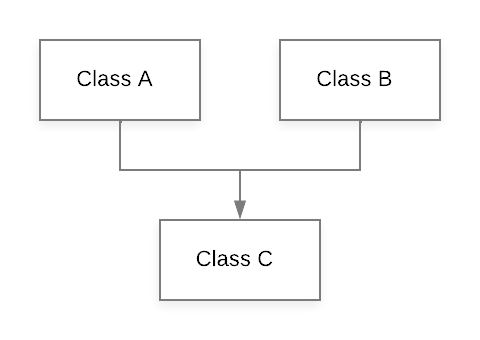
In the following Python code, the class C
is inheriting class A
and class C
and accessing their methods in the display
method:
class A:
def sayHi(self):
print("Hi")
class B:
def sayBye(self):
print("Bye")
class C(A, B):
def display(self):
super().sayHi()
super().sayBye()
c = C()
c.display()
Output:
Hello
World
3. Multilevel Inheritance
In multilevel inheritance, the child class inherits another child class. It is the chain of inheritance in which the parent class is the child of some other class.
The following block diagram illustrates an example of multilevel inheritance:
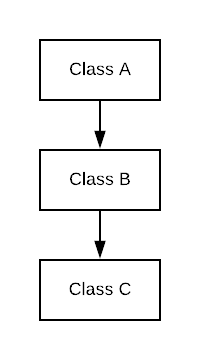
The multilevel inheritance is useful in representing multiple levels of ‘IS A’ relationships e.g. Animal is a Living being and Dog is an Animal (Here, living being, Animal, and Dog represent class A, class B, and class C respectively).
In the following Python example, class C
is accessing methods of its parent’s classes (i.e., class B
and class C
) through multilevel inheritance:
class A:
def display(self):
print("Class A")
class B(A):
def display(self):
super().display()
print("Class B")
class C(B):
def display(self):
super().display()
print("Class C")
c = C()
c.display()
Output:
Class A
Class B
Class C
4. Hierarchical Inheritance
Hierarchical inheritance is a type of inheritance where different multiple classes inherit a single parent class.
The following block diagram illustrates hierarchical inheritance:
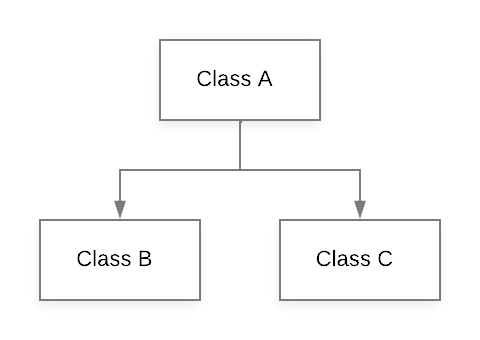
The hierarchical inheritance is useful in implementing class relationships where more than one class has an ‘IS A’ relationship with a single parent class e.g. Student is a Person and Employee is a Person (Here, Person is the parent class A whereas Student and Employee is class B and class C respectively).
Here is the Python implementation of the hierarchical inheritance where class B
and class C
both inherit class A
and calls its display
method:
class A:
def display(self, output):
print(output)
class B(A):
def display(self):
super().display('Hello from B')
class C(A):
def display(self):
super().display('Hello from C')
b = B()
b.display()
c = C()
c.display()
Output:
Hello from B
Hello from C
5. Hybrid Inheritance
Hybrid inheritance in Python is a combination of more than one type of inheritance. It is not any sequence or pattern of inheriting classes but is used as a naming convention where more than one type of inheritance is involved.
For example, in the following diagram, both single and multiple inheritance is taking place, thus forming hybrid inheritance:
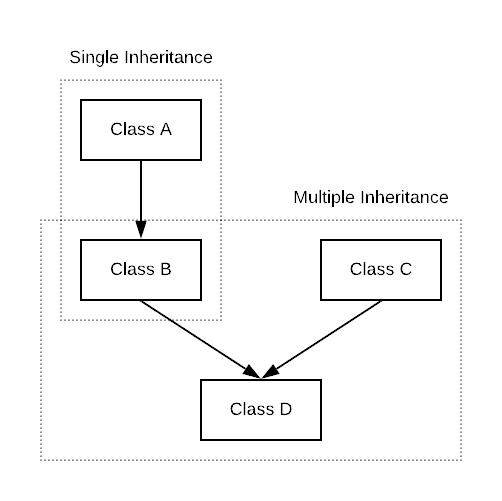
The following is another example of hybrid inheritance which has multilevel and single inheritance:
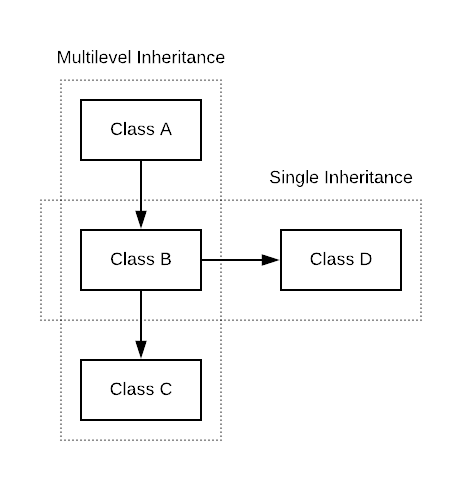
The Python implementation of this hybrid inheritance is as follows:
class A:
def display(self):
print("Super Parent display method")
""" class B used as intermediate class
to call class A's display method """
class B(A):
def display(self):
super().display()
''' child classes '''
class C(B):
def display(self):
super().display()
print("Class C display method")
class D(B):
def display(self):
super().display()
print("Class D display method")
c = C()
c.display()
d = D()
d.display()
Output:
Super Parent display method
Class C display method
Super Parent display method
Class D display method
Similar to this, different other types of inheritance can comprise a hybrid inheritance.
Thank you for providing this information I have read a lot of articles about this topic but none of giving clarity about inheritance.