Summary: In this tutorial, we will learn to read JSON from a File and write JSON to file using Python.
Example:
{
"lang" : "python",
"version" : 3,
"website" : "pencilprogrammer.com"
}
JSON (JavaScript Object Notation) is a lightweight format to interchange data. It is the text form of a javascript object. The key is of type “string “with double quotation marks and values can be string, number, nested JSON or array of the same.
JSON data is stored in JSON file (file with .json extension).
In Python, json.load()
and json.loads()
are the two methods that are used to read JSON data.
- json.load() reads JSON encoded data from a JSON file and converts it into a Python dictionary.
- json.loads() parse JSON string into a Python dictionary.
We should not get confused between the two methods. The json.load()
method is used to read data from a .json file whereas the json.loads()
method is used to read JSON data in the form of a string.
Example: Read JSON File in Python
import json
#open json file
data_file = open('data.json')
'''
parse json encoded data into dictionary
using json.load()
'''
data_dict= json.load(data_file)
print(data_dict)
Output:
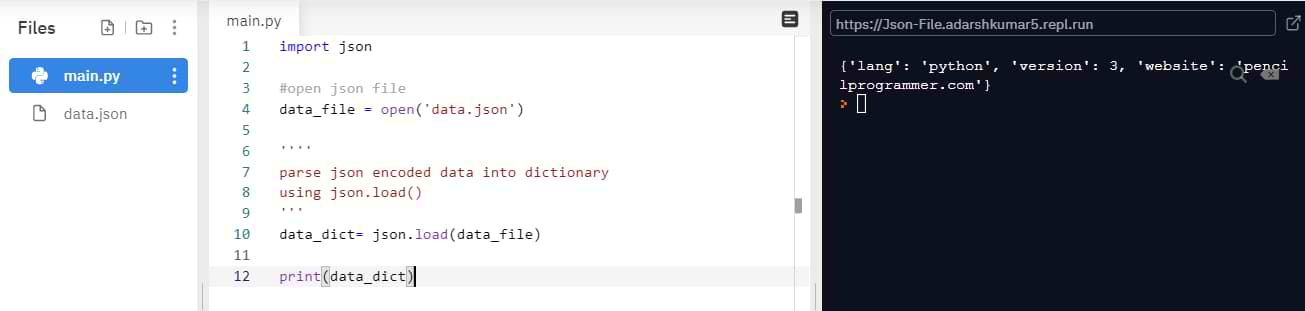
The data.json
contains the encoded JSON data. We first load it into our program, then parse it into a dictionary using the json.load()
method.
Example: Read JSON String in Python
In the above example, we read a JSON file. In this example, we will read a JSON string and parse it into a dictionary using json.loads()
.
import json
#JSON String
json_string = '{ "website" : "pencilprogrammer.com" }'
#JSON data into dictionary
data_dict= json.loads(json_string)
print(data_dict)
Output:
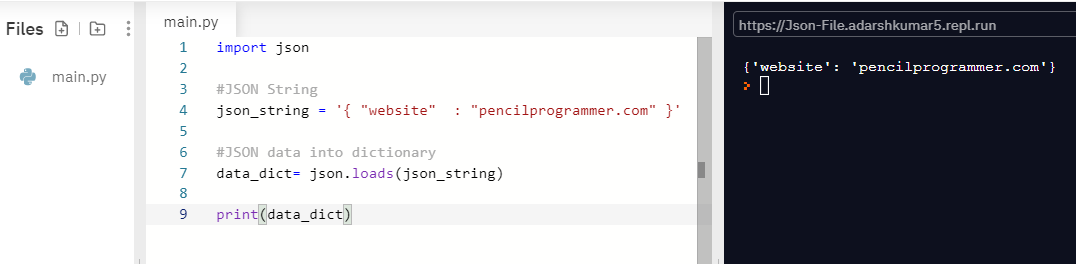