Problem: Write a C program to check whether the number is Neon or not.
A number is called a Neon Number if the sum of digits of the square of the number is equal to the original number.
Example: 9
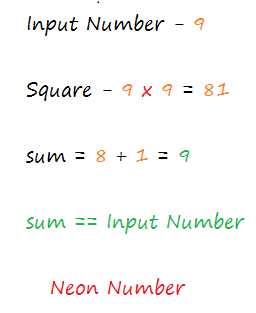
Steps to check neon number in C:
- Input a number.
- Calculate the square of the number.
- Sum digits of the square.
- Check whether the calculated sum is equal to the original number.
- If yes then the number is Neon, else it is not.
Here is the implementation of the steps in C:
#include <stdio.h>
int main()
{
int num, digit, sum =0;
//Input a number
printf("Enter a number to check for neon number: \n");
scanf("%d",&num);
//Calculate square of the input
int square = num*num;
//Sum the digits of the square
while(square!=0){
digit = square%10;
sum += digit;
square = square/10;
}
//Check if sum is equal to the input number
if(sum == num)
printf("Neon Number \n");
else
printf("Not a Neon Number \n");
return 0;
}
Output:
Enter a number to check for neon number:9
Neon Number
To sum up the digits of the square, we have individually extracted each digit using the Modulus operator (i.e. square%10
) and added it to the sum
variable.
In the end, we compared the calculated sum
with the input number num
to check whether the given number is a Neon number or not.
I appreciate your work