Summary: In this programming example, we will learn how to multiply two matrices in C++.
Example:
m1 = {{1, 2, 3}, {1, 1, 1}} m2 = {{1, 2}, {1, 3}, {1, 1}} m1*m2 = {{6, 11}, {3, 6}}
Condition for Matrix Multiplication
Two matrices A and B can be multiplied only if the number of columns in A is equal to the number of rows in B.
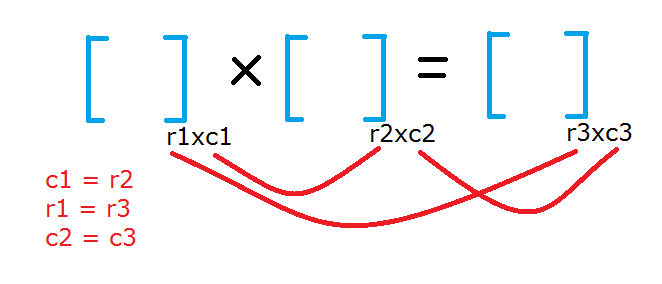
Also, the order of the resultant matrix is as follows:
- row = number of rows in the first matrix.
- columns = number of columns in the second matrix.
So before multiplying, first we verify the order of the given matrix and then define the order for the resulting matrix accordingly.
Algorithm to Multiply Two Matrix in C++
- Loop (
I
) from 0 to row order of the first matrix.- Nest (
J
) another loop from 0 to the column order of the second matrix.- Nest another loop (K) from 0 to row order of the second matrix.
- Sum
(matrix1[I][K] * matrix2[K][J])
- Sum
- Store the final sum into the resultant matrix as
res[I][J] = sum
.
- Nest another loop (K) from 0 to row order of the second matrix.
- End (
J
) loop.
- Nest (
- End (
I
) loop.
Basically, we have to nest 3 ‘for’ loops in order to multiply and store the multiplication result into the resultant matrix.
Here is the C++ example that multiply two given matrices:
#include <iostream>
using namespace std;
int main()
{
//order of matrix A
int r1 = 2, c1 = 3;
//order of matrix B
int r2 = 3, c2 = 2;
//matrix A
int m1[r1][c1] = {{1, 2, 3},
{1, 1, 1}};
//matrix B
int m2[r2][c2] = {{1, 2},
{1, 3},
{1, 1}};
//Check order of Matrix A and B
if(c1 != r2){
cout << "Matrix multiplaction Not possible for the given matrices";
} else {
//define order of the resultant matrix
int res[r1][c2];
//Multiply the elements of A and B
for(int i=0; i<r1; i++){
for(int j=0; j<c2; j++){
int sum =0;
for(int k=0; k<r2; k++){
sum += (m1[i][k] * m2[k][j]);
}
res[i][j] = sum;
}
}
//output the resultant matrix
cout << "Resultant Matrix: \n";
for(int i=0; i<r1; i++){
for(int j=0; j<c2; j++){
cout << res[i][j] << "\t";
}
cout << "\n";
}
}
return 0;
}
Output:
Resultant Matrix6 11
3 6
In this tutorial, we learned to multiply matrices in the C++ programming language.