Problem: Write a Java program to check whether the given number is Special or not.
A number is said to be special if the sum of the factorial of its digits is equal to the original number. For example: 145.
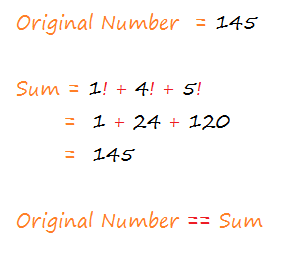
Steps to check special number in Java:
- Input a number.
- Find the factorial of its digits.
- Add all the factorials.
- Check if the computed sum is equal to the original number.
- If so then the number is special, otherwise not.
Here is the java program that implement the above steps to check special number:
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner in=new Scanner(System.in);
int digit, n, sum=0, temp;
//1. Input the number.
System.out.println("Enter a number");
n = in.nextInt();
temp = n;
//2. Find the factorial of its digits.
//3. Add all the factorials.
while(temp != 0){
digit = temp%10;
sum += factorial(digit);
temp = temp/10;
}
//4. Check if the computed sum is equal to the original number.
if(sum == n)
System.out.println("Special number");
else
System.out.println("Not a Special Number");
}
//function to compute the factorial of the digits
private static int factorial(int num){
int f=1;
for(int i=2; i<=num; i++){
f = f*i;
}
return f;
}
}
Output:
Enter a number
145
Special number
Print all Special Numbers in the Range
To print all special numbers, we individually check each number in the given range whether it is special or not, and output them accordingly.
For this, we write the logic to check special number (same as used in the above program) in a separate function and use it for all numbers in the given interval.
import java.util.Scanner;
class Main {
//Array with factorials of 0 to 9
static int factorial[] = {1, 1, 2, 6, 24, 120, 720, 5040, 40320, 362880};
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
boolean hasSpecial = false;
//Enter the range values
System.out.print("Enter the lower and upper value of the Interval: ");
int lower = in.nextInt();
int upper = in.nextInt();
//Iterate through all numbers in the range
for(int i=lower; i<=upper; i++){
//use isSpecial() method to check if it is special
if(isSpecial(i)){
System.out.print(i+" ");
hasSpecial = true;
}
}
//If the range doesn't have any special number
if(!hasSpecial)
System.out.println("No special numbers were found in the given range");
}
//function returns true if the passed number is special
private static boolean isSpecial(int n){
int digit, sum=0, temp= n;
//Extract digits and sum their factorial
while(temp != 0){
digit = temp%10;
sum += factorial[digit];
temp = temp/10;
}
return sum == n;
}
}
Output:
Enter the lower and upper value of the Interval: 1 1000
1 2 145
In this programming example, we learned to check number using the Java programming language.
Plz write a program to print first n special numbers