Problem: Write a Python program to check whether the given number is Automorphic or not.
An automorphic number is a number whose square ends in the same digits as the original number itself. Examples: 5, 76, etc.
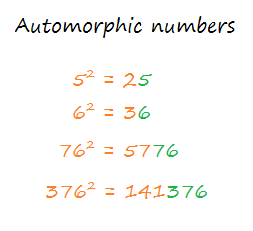
To check whether a number is automorphic or not:
- Input a number.
- Count the digits in the input number (n).
- Compute the square of the number.
- Extract the last ‘n’ digits from the square and check if it is same as the original number.
- If so then the given number is Automorphic, otherwise not.
num = int(input("Enter a number: \n"))
#find number of digits in num
n = len(str(num))
#compute square
sqr = num**2
#Extract last n digits from the square
last = sqr%pow(10,n)
#compare last n digits with the input number
if last == num:
print("Automorphic Number")
else:
print("Not an Automorphic Number")
Output:
Enter a number: 76
Automorphic Number
Find all Automorphic numbers in the Given Interval
To find and print all Automorphic numbers, we will check each number of the interval using the same logic as used in the above program.
For this, we will write the logic to check the Automorphic number in a different function and call the same function for every number using a for loop.
#function returns true if num is Automorphic otherwise false def isAutomorphic(num): #square of the number sqr = num**2 #number of digits in num n = len(str(num)) #last n digits of the square last = sqr%pow(10,n) return last == num low, high = [int(x) for x in input("Enter a lower and upper Range value: \n").split()] #loop through all the numbers in the range for x in range(low,high): if isAutomorphic(x): print(x,end=' ')
Output:
Enter a lower and upper Range value: 1 100
1 5 6 25 76