Problem: Write a Python program to multiply two matrices and output the resultant matrix.
Example:
m1 = [[8,1], [2,1]] m2 = [[8,1], [2,1]] m1*m2 = [[25,10,17], [7, 4, 5]]
A matrix in python can be represented using a nested list.
For example, m1=[[8,1], [2,1]]
is a matrix of order (2×2).
The value of len(m1)
and len(m1[0])
are the number of rows and columns in the matrix respectively.
Also, two matrices can only be multiplied if the number of columns of the first matrix is equal to the number of rows of the second matrix.
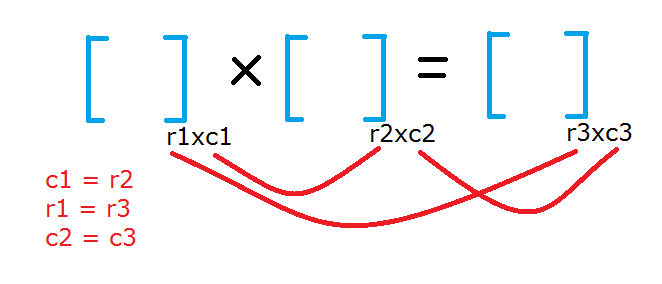
The order of the resultant matrix is the number of rows of the first matrix and the number of columns of the second matrix.
Knowing all these, we write the code in python to multiply matrices implementing the standard rule in mathematics.
Method 1: Using Nested Loop
Recommended:
#Matrix I
m1 = [[8,1],
[2,1]]
#Matrix II
m2 = [[3,1,2],
[1,2,1]]
#resultant matrix
res = [[0,0,0],
[0,0,0]]
#orders ot the two input matrices
r1 = len(m1)
c1 = len(m1[0])
r2 = len(m2)
c2 = len(m2[0])
'''
check if matrices can be multiplited or not,
If so, then multiply otherwise output the appropiate message.
'''
if c1 != r2:
print("Matrix Multiplication not possible for given orders")
else:
for i in range(r1):
for j in range(c2):
sum = 0
for k in range(c1):
sum += (m1[i][k] * m2[k][j])
res[i][j] = sum
#output the resultant matrix
print(res)
Output:
[[25, 10, 17], [7, 4, 5]]
Method 2: Using List Comprehension
This method is the modified form of the above program. The only difference is that the nested loop is replaced by the list comprehension technique in python.
#Matrix I
m1 = [[8,1],
[2,1]]
#Matrix II
m2 = [[3,1,2],
[1,2,1]]
#orders of the two input matrices
r1 = len(m1)
c1 = len(m1[0])
r2 = len(m2)
c2 = len(m2[0])
if c1 != r2:
print("Matrix Multiplication not possible for given orders")
else:
res = [[sum((m1[i][k] * m2[k][j]) for k in range(c1)) for j in range(c2)] for i in range(r1)]
#output the resultant matrix
print(res)
Output:
[[25, 10, 17], [7, 4, 5]]
The use of list comprehension may be a little difficult to understand but if looked carefully, you will notice that it is the simplified version of the nested ‘for loop’.
If you have any doubts or suggestions then please comment below.