Summary: In this tutorial, we will learn what are pointers in Python, how they work and do they even exist in Python? You’ll gain a better understanding of variables and pointers in this article.
What are the Pointers?
Pointers in the context of high-level languages like C++ or Java are nothing but variables that stores the memory address of other variables or objects.
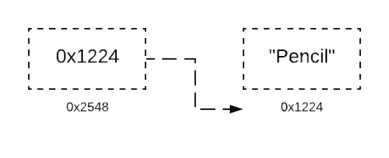
We can reference another variable stored in a different memory location using a pointer in high-level programming languages.
Unlike variables, pointers deal with address rather than values.
Does Python have Pointers?
Sadly, Python doesn’t have pointers like other languages for explicit use but is implemented under the hood.
Types such as list, dictionary, class, and objects, etc in Python behave like pointers under the hood.
The assignment operator =
in Python automatically creates and assigns a pointer to the variable.
Example:
l = [1,2,3,4]
The above statement creates a list object and points a pointer to it called “l”.
If you assign the same list object both to two different variables m and l, they will point to the same memory location.
#Assign
l = [1,2,3,4]
m = l
#Modify only m
m[0] = 9
#changes reflected by both m and l
print("m =", m)
print("l =", l)
Output:

To make sure that m and l are pointing to same object lets use is
operator.
>>> l = [1,2,3,4]
>>> m = l
>>> print(m is l)
True
Python “is” operator works differently from “==” operator. “==” operator in Python returns compares the content of the object whereas the is operator compares the object’s reference.
Also, it is important to note that python deletes an object only when all the pointers to the object is deleted.
Use del m
or del l
will only delete the variable not the underlying object to which it was referencing.
Python will still keep the object in the memory until all the pointers pointing to the list object are deleted.
Are numbers and strings also Pointers?
The answer is NO. Simples types such as int
, str
, float
, bool
, etc do not behave like a pointer.
Consider the following python snippet:
#Declare variables
a = 9
b = a
#modifying a
a = 2
print("a =",a)
print("b =",b)
Output:

As we can see, changing the value of “a” doesn’t change “b”. Hence unlike a list, the value of integer variables a
and b
are stored in different memory addresses or locations.
On a final note, we conclude that Python doesn’t have pointers but the behavior of pointers is implemented under the hood by the python for a few types and objects.