Problem: Write a Program in C to output the transpose of a matrix.
Transpose of the matrix is converting the rows into columns and columns into the rows. Whether a matrix is a square or rectangle its transpose is possible.
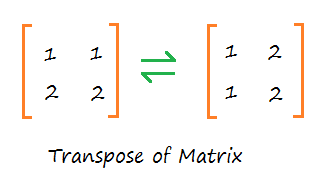
To find the transpose of a matrix in C, we need to iterate through columns before iterating through row i.e. ‘for loop’ for row iteration should be nested inside the ‘for loop’ for column iteration.
#include <stdio.h>
#define row 4 // Change for different row value
#define col 4 // Change for different column value
int main()
{
//2D Matrix
int matrix[row][col] = {{1, 1, 1, 1},
{2, 2, 2, 2},
{3, 3, 3, 3},
{4, 4, 4, 4}};
printf("Original Matrix: \n");
for(int i=0; i<row; i++){
for(int j=0; j<col; j++){
printf("%d ",matrix[i][j]);
}
printf("\n");
}
/*
*Display the transpose by first iterating through-
*column index then nested iterating row index
*/
printf("Transpose of Matrix: \n");
for(int j=0; j<col; j++){
for(int i=0; i<row; i++){
printf("%d ",matrix[i][j]);
}
printf("\n");
}
return 0;
}
Output:
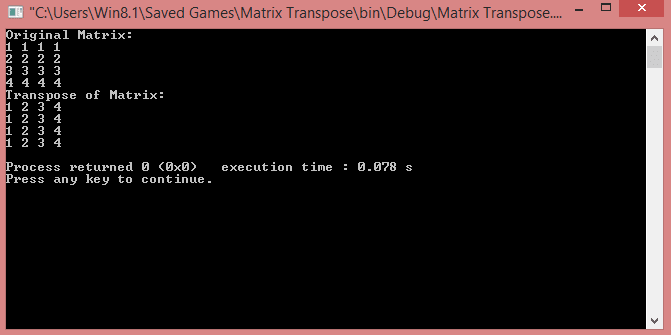
In the above program, we have defined the order of the matrix (i.e. number of rows and columns) using the preprocessor define
command.
We declared the matrix, initialized it with the required elements and then printed the transpose of it using the described algorithm.
In this tutorial, we learned to transpose a matrix in the C programming language. If you have any doubts or suggestions then please comment below.